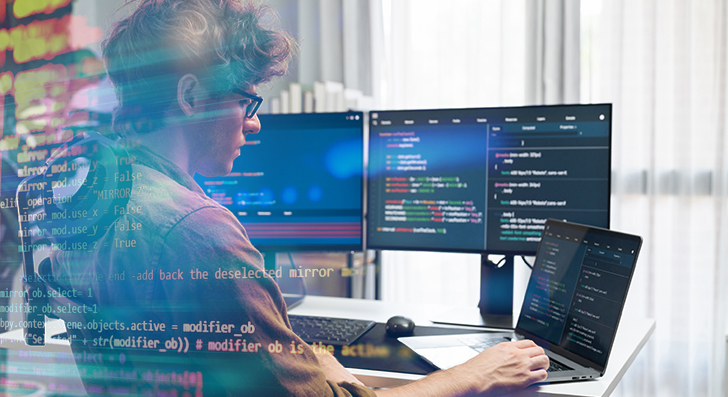
Scalability implies your software can take care of progress—much more people, far more info, and even more traffic—without breaking. For a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Numerous apps fail if they develop rapid simply because the initial style can’t tackle the additional load. As being a developer, you might want to think early regarding how your method will behave under pressure.
Get started by developing your architecture to generally be flexible. Steer clear of monolithic codebases the place everything is tightly linked. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial components. Every single module or company can scale on its own without having impacting The complete program.
Also, contemplate your database from working day one. Will it require to deal with 1,000,000 buyers or just a hundred? Choose the proper form—relational or NoSQL—according to how your info will increase. Plan for sharding, indexing, and backups early, even if you don’t need to have them nevertheless.
A different important point is to prevent hardcoding assumptions. Don’t generate code that only is effective under current circumstances. Take into consideration what would take place When your consumer base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use layout designs that help scaling, like concept queues or occasion-driven systems. These help your application deal with much more requests with out obtaining overloaded.
Whenever you build with scalability in your mind, you are not just planning for success—you're reducing potential headaches. A effectively-planned system is less complicated to keep up, adapt, and expand. It’s much better to arrange early than to rebuild afterwards.
Use the ideal Databases
Selecting the right databases can be a important part of making scalable apps. Not all databases are constructed the same, and using the Erroneous one can gradual you down as well as result in failures as your application grows.
Start off by comprehending your data. Could it be extremely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with relationships, transactions, and consistency. In addition they assist scaling strategies like examine replicas, indexing, and partitioning to deal with additional site visitors and details.
When your data is much more adaptable—like user action logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more conveniently.
Also, contemplate your read and publish styles. Are you currently undertaking lots of reads with fewer writes? Use caching and browse replicas. Will you be handling a large produce load? Explore databases which will handle higher publish throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Imagine forward. You might not will need Highly developed scaling features now, but selecting a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases effectiveness while you increase.
In short, the right databases depends on your application’s composition, velocity desires, And just how you assume it to increase. Get time to choose correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your app grows, each and every little delay provides up. Improperly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Option if an easy one will work. Maintain your capabilities small, targeted, and straightforward to check. Use profiling equipment to find bottlenecks—destinations in which your code takes far too extended to operate or makes use of too much memory.
Upcoming, examine your database queries. These generally slow points down greater than the code alone. Make certain Each individual query only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
Should you detect exactly the same knowledge getting asked for again and again, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application additional efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may crash every time they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These methods support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more end users plus much more website traffic. If all the things goes as a result of just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of just one server undertaking every one of the perform, the load balancer routes people to diverse servers depending on availability. This means no one server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it might be reused promptly. When consumers request the exact same data again—like an item webpage or a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces databases load, enhances velocity, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does modify.
Briefly, load balancing and caching are easy but powerful equipment. Alongside one another, they help your app cope with more end users, continue to be quick, and Recuperate from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you need resources that allow your read more application mature easily. That’s in which cloud platforms and containers are available. They give you versatility, lessen set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to rent servers and expert services as you would like them. You don’t have to purchase hardware or guess potential capability. When targeted traffic will increase, you may insert additional means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you can scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You are able to concentrate on developing your app in lieu of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This makes it easy to maneuver your app in between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one part within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools indicates you may scale rapid, deploy effortlessly, and Get better rapidly when challenges take place. If you prefer your app to improve with out boundaries, start employing these applications early. They conserve time, lower danger, and allow you to continue to be focused on constructing, not correcting.
Keep track of All the things
Should you don’t watch your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot concerns early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking primary metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Regulate how much time it takes for users to load pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s occurring within your code.
Put in place alerts for critical challenges. One example is, When your reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified immediately. This helps you take care of challenges rapid, generally ahead of consumers even recognize.
Monitoring can also be useful when you make modifications. If you deploy a completely new element and see a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, traffic and facts boost. With out checking, you’ll overlook indications of difficulties until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you'll be able to Make apps that mature smoothly devoid of breaking stressed. Commence smaller, think massive, and Establish intelligent.